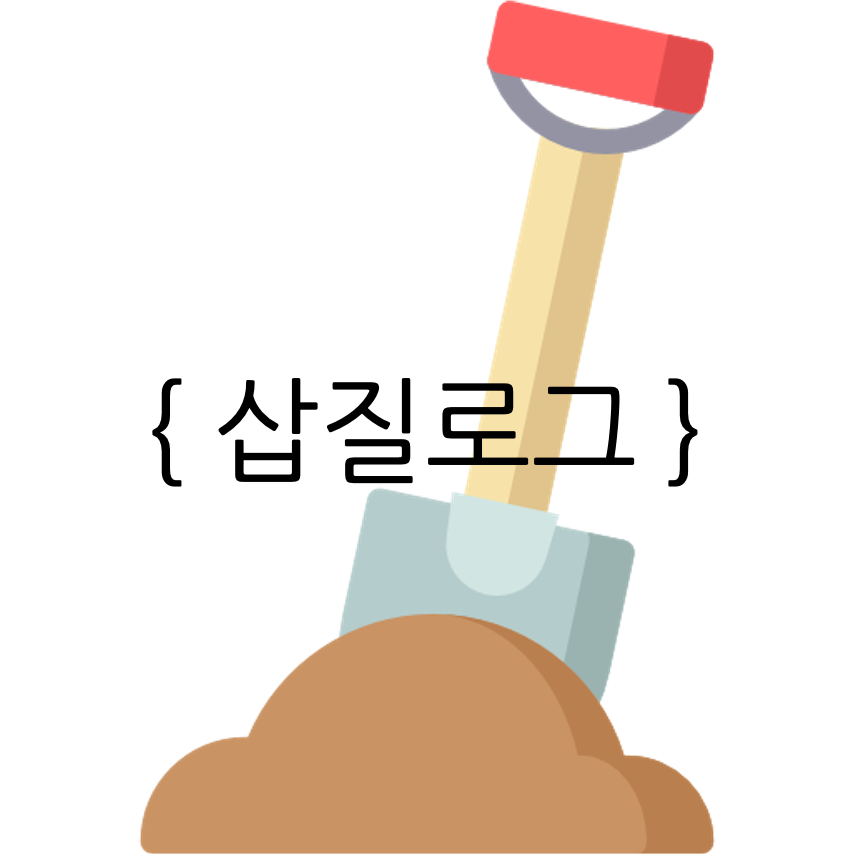
문제상황
지난 삽질로그에서 계속 고민하던 문제인 '왜 지연로딩을 설정했는데도 참조하고 있는 객체를 통째로 들고다니지?' 라는 물음에 대한 이유를 어느정도 파악한 것 같다.
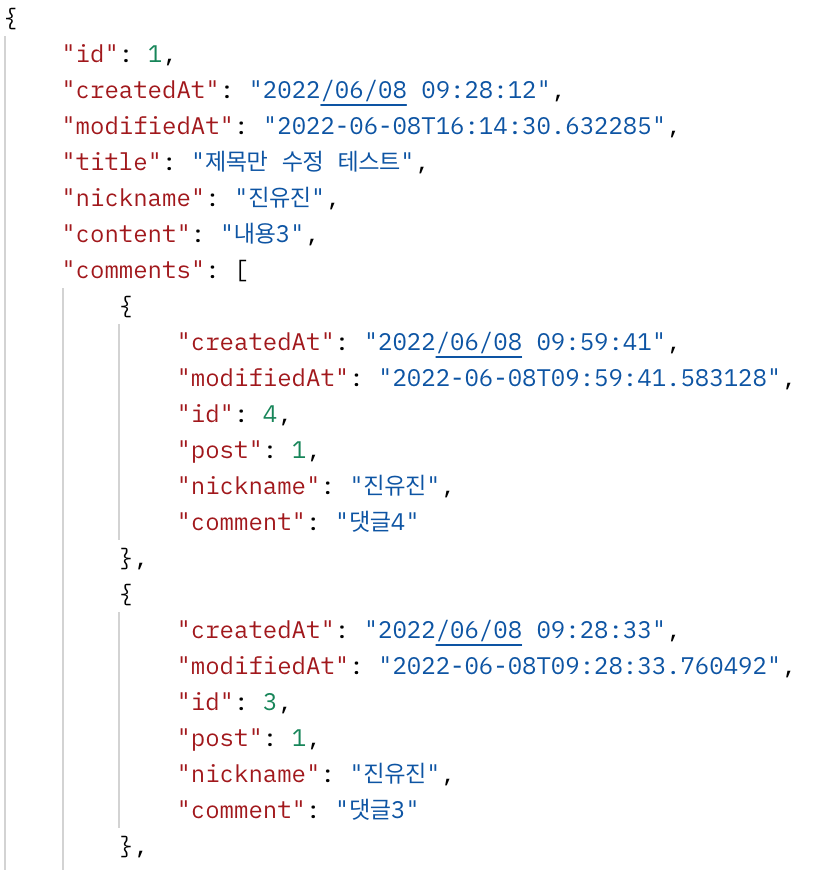
PostRestController.java
@GetMapping("")
public List<Post> getPosts() {
List<Post> postsList = postService.getPosts();
return postsList;
}
PostService.java
public List<Post> getPosts() {
List<Post> posts = postRepository.findAllByOrderByCreatedAtDesc();
return posts;
}
Post.java
@Entity
@Getter
@NoArgsConstructor
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id")
public class Post extends Timestamped{
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String nickname;
@Column(nullable = false)
private String content;
@OrderBy(value = "createdAt DESC")
@OneToMany(mappedBy = "post", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Comment> comments;
Comment.java
@Entity
@Getter
@NoArgsConstructor
public class Comment extends Timestamped{
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "POST_ID", nullable = false)
private Post post;
@Column(nullable = false)
private String nickname;
@Column(nullable = false)
private String comment;
해결과정
Comment Entity를 보면 제대로 지연로딩을 설정해두었다.
PostRestController를 타고 들어가면서 문제가 생겼으므로 PostService를 테스트 코드에 넣어서 쿼리문을 확인해보면
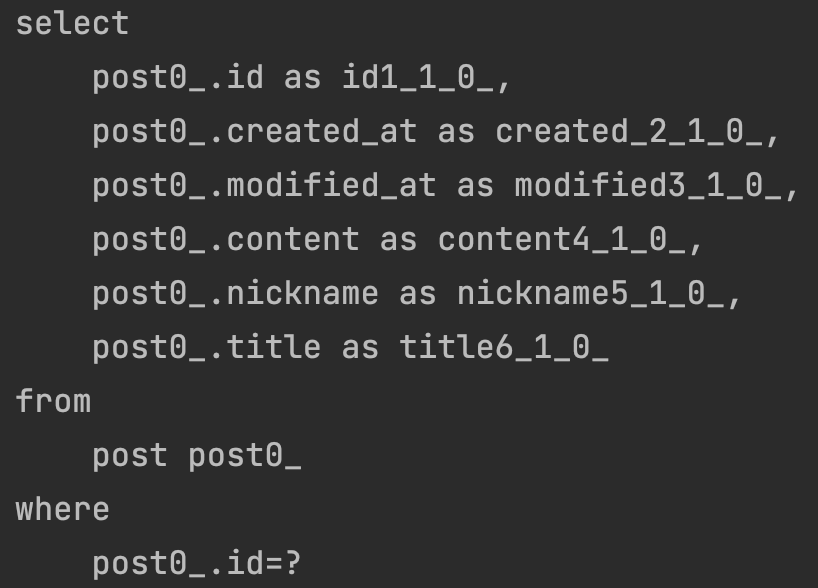
Post Entity만 조회하고 내가 생각했던대로 Comment Entity는 조회하지 않는게 확인된다.
그런데 왜 Controller에서 조회를 하면 참조 객체를 다 들고오는걸까?
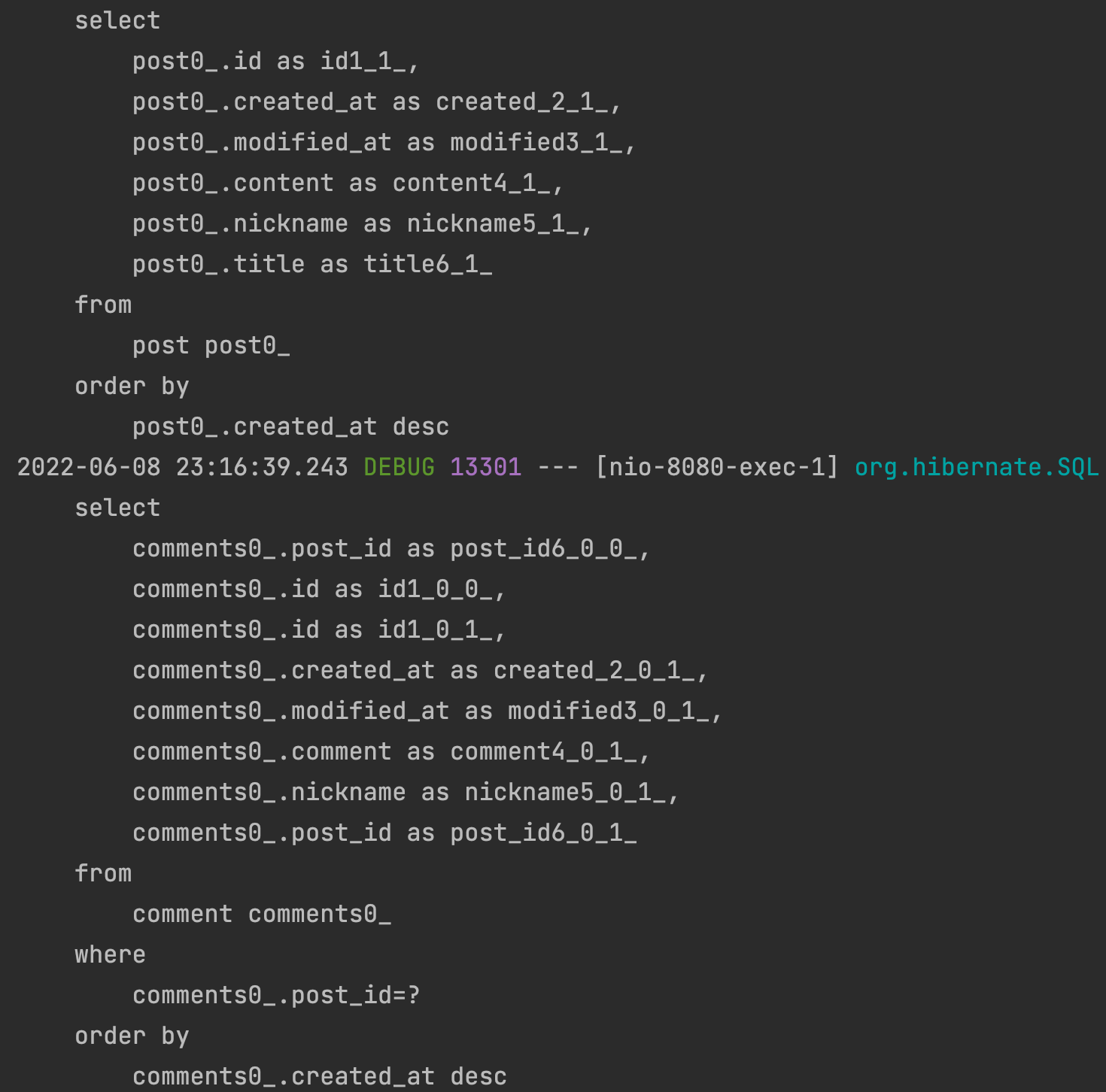
getPosts를 수행하고 나면 Post와 Comment를 둘다 조회하는 쿼리문이 날아가는걸 확인할 수 있다.
잘 생각해봤을 때, PostRestController에서 Post Entity를 통째로 반환하기 때문에 Post가 참조하고 있는 Comment 객체를 함께 직렬화해서가 아닐까?
확인을 위해 Entity대신 응답용 DTO를 생성해서 매핑 후 return해보기로 했다.
PostResponseDto.java
@Getter @Setter
public class PostResponseDto {
private Long id;
private String title;
private String nickname;
private String content;
pirvate LocalDateTime createdAt;
}
PostService.java
public List<PostResponseDto> getPosts() {
List<Post> posts = postRepository.findAllByOrderByCreatedAtDesc();
ModelMapper modelMapper = new ModelMapper();
List<PostResponseDto> postList = new ArrayList<>();
for (Post post : posts) {
PostResponseDto mappedPosts = modelMapper.map(post, PostResponseDto.class);
postList.add(mappedPosts);
}
return postList;
}
간단한 매핑을 위에 modelMapper 라이브러리를 사용해서 Entity to DTO 매핑 후 테스트를 해보았다.

지연로딩 설정에 따라 comment 객체 조회쿼리는 날아가지 않는 걸 확인할 수 있다.
즉, 원인은 Controller에서 Entity를 통째로 리턴하려고 하는 데에 있었다. 현재는 기획상 post가 comment 객체도 다 불러와야해서 entity를 통째로 반환하는 방식을 사용하고 있지만 추후에 프로젝트를 하게 된다면 응답용 DTO를 설계해서 사용하도록 해야겠다.
'Study > Trouble Shooting' 카테고리의 다른 글
[삽질로그] CORS 정책 위반 (0) | 2022.06.19 |
---|---|
[삽질로그] 소스트리 강제 종료 에러 (0) | 2022.06.11 |
[삽질로그] Entity 관련 삽질 (0) | 2022.06.08 |
[삽질로그] 깃 잔디가 안심어지는 문제 (0) | 2022.05.16 |
[삽질로그] 파이썬 missing 1 required positional argument: 에러 (0) | 2022.05.15 |
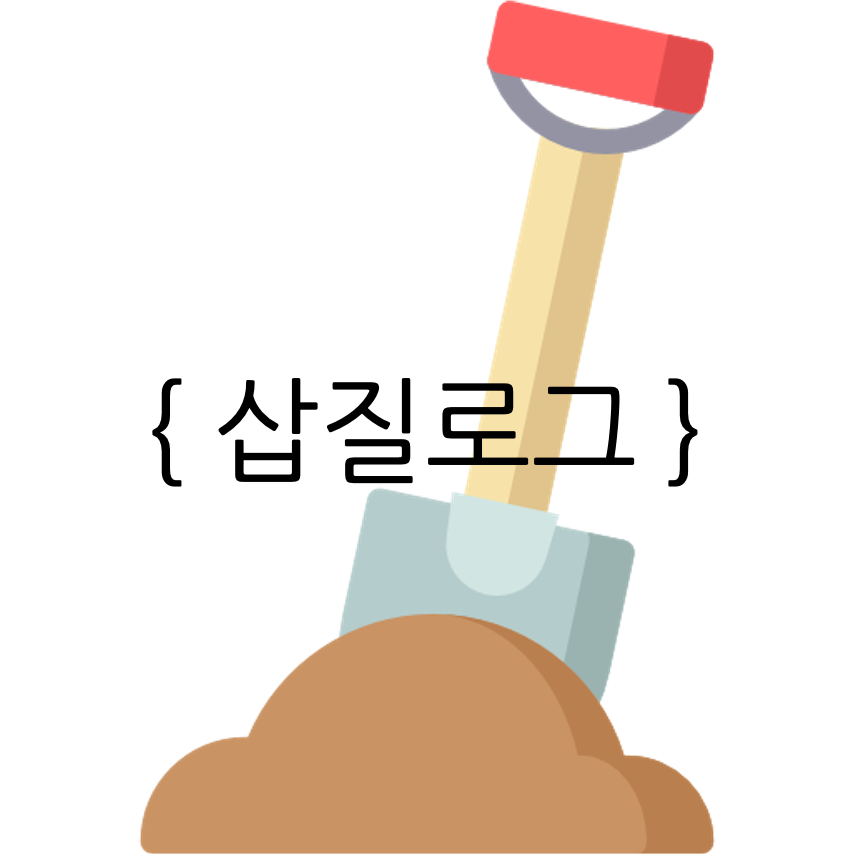
문제상황
지난 삽질로그에서 계속 고민하던 문제인 '왜 지연로딩을 설정했는데도 참조하고 있는 객체를 통째로 들고다니지?' 라는 물음에 대한 이유를 어느정도 파악한 것 같다.
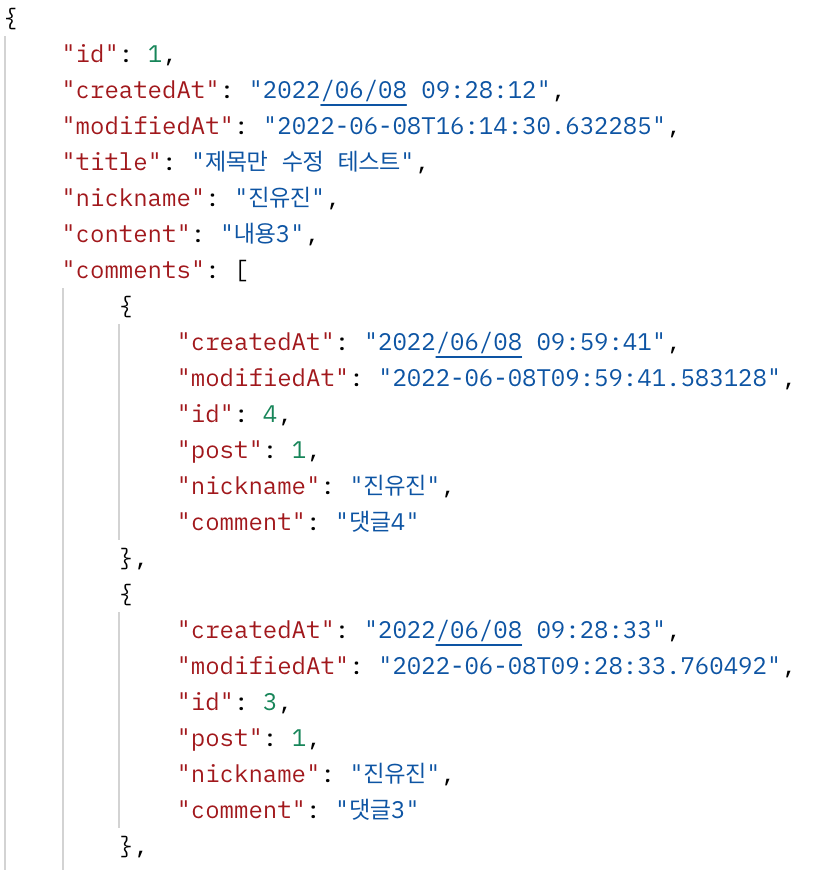
PostRestController.java
@GetMapping("")
public List<Post> getPosts() {
List<Post> postsList = postService.getPosts();
return postsList;
}
PostService.java
public List<Post> getPosts() {
List<Post> posts = postRepository.findAllByOrderByCreatedAtDesc();
return posts;
}
Post.java
@Entity
@Getter
@NoArgsConstructor
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id")
public class Post extends Timestamped{
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String nickname;
@Column(nullable = false)
private String content;
@OrderBy(value = "createdAt DESC")
@OneToMany(mappedBy = "post", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Comment> comments;
Comment.java
@Entity
@Getter
@NoArgsConstructor
public class Comment extends Timestamped{
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "POST_ID", nullable = false)
private Post post;
@Column(nullable = false)
private String nickname;
@Column(nullable = false)
private String comment;
해결과정
Comment Entity를 보면 제대로 지연로딩을 설정해두었다.
PostRestController를 타고 들어가면서 문제가 생겼으므로 PostService를 테스트 코드에 넣어서 쿼리문을 확인해보면
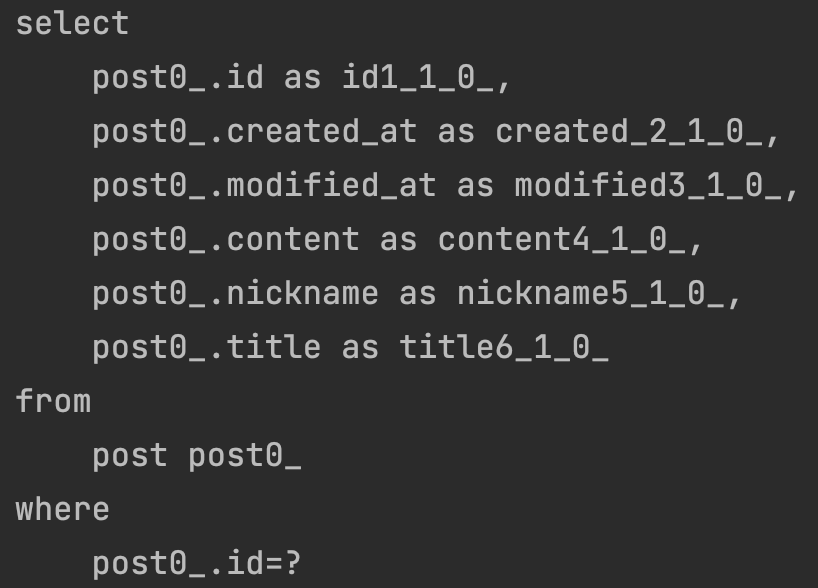
Post Entity만 조회하고 내가 생각했던대로 Comment Entity는 조회하지 않는게 확인된다.
그런데 왜 Controller에서 조회를 하면 참조 객체를 다 들고오는걸까?
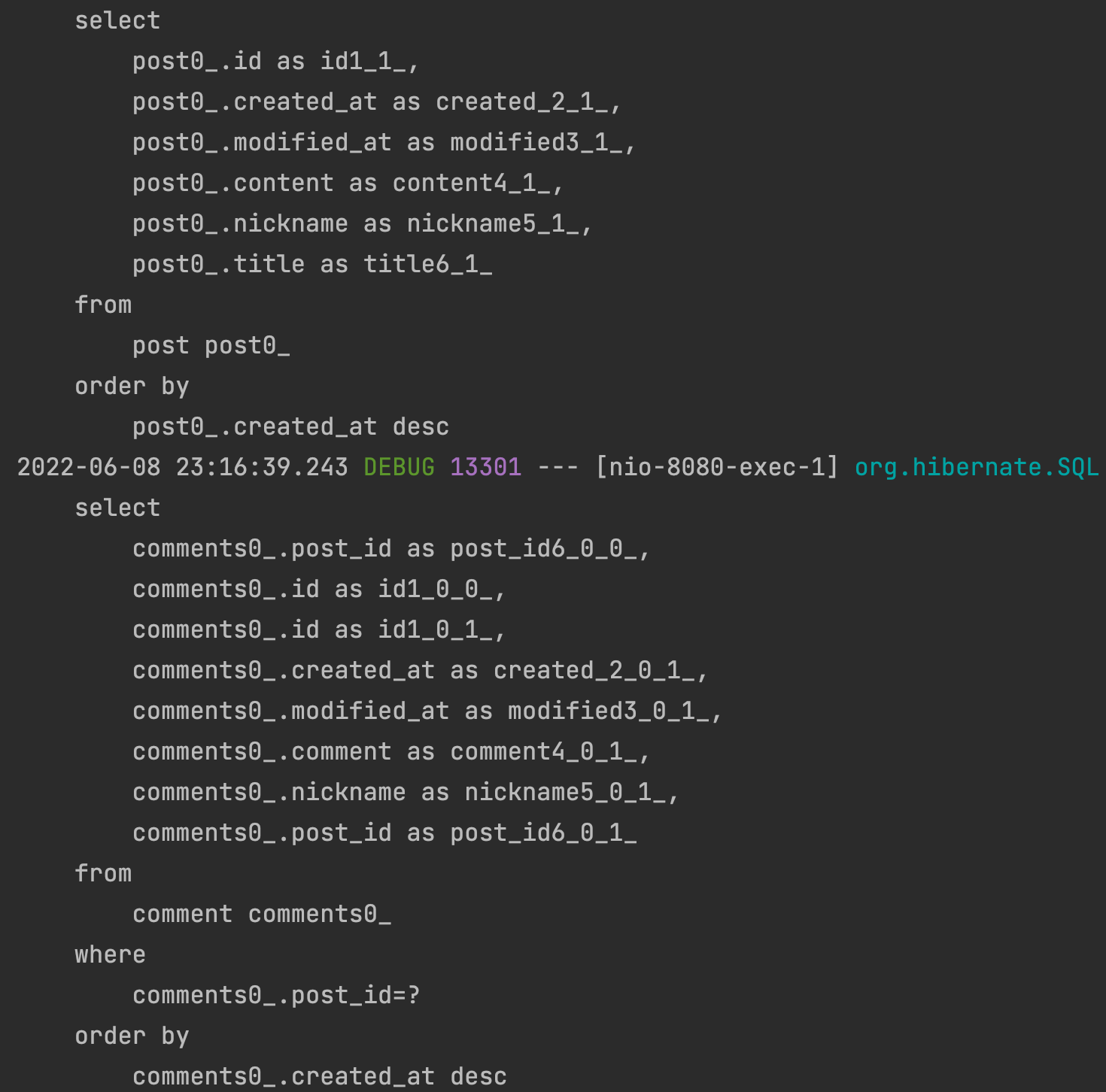
getPosts를 수행하고 나면 Post와 Comment를 둘다 조회하는 쿼리문이 날아가는걸 확인할 수 있다.
잘 생각해봤을 때, PostRestController에서 Post Entity를 통째로 반환하기 때문에 Post가 참조하고 있는 Comment 객체를 함께 직렬화해서가 아닐까?
확인을 위해 Entity대신 응답용 DTO를 생성해서 매핑 후 return해보기로 했다.
PostResponseDto.java
@Getter @Setter
public class PostResponseDto {
private Long id;
private String title;
private String nickname;
private String content;
pirvate LocalDateTime createdAt;
}
PostService.java
public List<PostResponseDto> getPosts() {
List<Post> posts = postRepository.findAllByOrderByCreatedAtDesc();
ModelMapper modelMapper = new ModelMapper();
List<PostResponseDto> postList = new ArrayList<>();
for (Post post : posts) {
PostResponseDto mappedPosts = modelMapper.map(post, PostResponseDto.class);
postList.add(mappedPosts);
}
return postList;
}
간단한 매핑을 위에 modelMapper 라이브러리를 사용해서 Entity to DTO 매핑 후 테스트를 해보았다.

지연로딩 설정에 따라 comment 객체 조회쿼리는 날아가지 않는 걸 확인할 수 있다.
즉, 원인은 Controller에서 Entity를 통째로 리턴하려고 하는 데에 있었다. 현재는 기획상 post가 comment 객체도 다 불러와야해서 entity를 통째로 반환하는 방식을 사용하고 있지만 추후에 프로젝트를 하게 된다면 응답용 DTO를 설계해서 사용하도록 해야겠다.
'Study > Trouble Shooting' 카테고리의 다른 글
[삽질로그] CORS 정책 위반 (0) | 2022.06.19 |
---|---|
[삽질로그] 소스트리 강제 종료 에러 (0) | 2022.06.11 |
[삽질로그] Entity 관련 삽질 (0) | 2022.06.08 |
[삽질로그] 깃 잔디가 안심어지는 문제 (0) | 2022.05.16 |
[삽질로그] 파이썬 missing 1 required positional argument: 에러 (0) | 2022.05.15 |